An ode to console.log()
When console.log is the best way you learned how to debug, it looks like a decent solution for most problems. Read: "Everything Starts Out Looking Like a Toy" #165
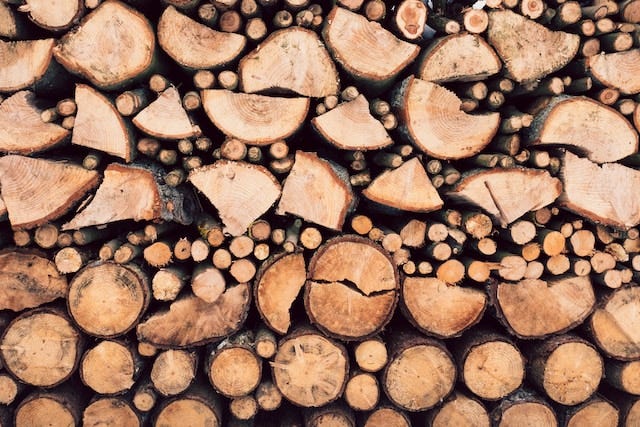
Hi, I’m Greg 👋! I write weekly product essays, including system “handshakes”, the expectations for workflow, and the jobs to be done for data. What is Data Operations? is a post that grew into Data & Ops, a team to help you with product, data, and operations.
This week’s toy: converting a junk car into a working generator. It turns out that an engine, an alternator, and some Arduino hacking can create clean AC power to run your stuff. You’ll be better prepared for the zombie apocalypse now - or maybe just for the next winter storm. Edition 165 of this newsletter is here - it’s October 2, 2023.
If you have a comment or are interested in sponsoring, hit reply.
The Big Idea
A short long-form essay about data things
⚙️ An ode to console.log()
Some of the first programs I ever wrote on a computer used PRINT to echo a line to the screen. Using BASIC, I filled the view with something like:
10 PRINT “This is cool!”
20 PRINT GOTO 10
Yep, garden variety “hello world” in BASIC. It’s a lot of years later and I am still relying on a simple function to debug and write text to the screen. In this case, I am a person who uses console.log() a lot when developing Javascript or other code.
Console.log – if you’re not familiar with it – is literally a print function that can output information to the browser console. If you want, you can use it on any web site to send yourself a message in the console or output information that’s present in a page.
That means when you’re building some code – let’s say a loop or other kind of control structure where you make a request – and you want to make sure you know what’s going on when the code actually runs, you might use console.log to find out what’s happening.
Walk along with the code as it’s happening
What should you log? If something significant happened, you might want to log before and after that thing happened. It’s tempting to be careless and write “something happened” or “I got to here and then the code errored” but future you will not be happy with this lack of context when you need to read your own code months later.
I am here to tell you: that there are better ways to use console.log (some of which I learned even this week!)
There are a few things you can do in particular that will help improve the readability and usefulness of your code:
While working, use pseudo-code to check your logic - you don’t need to do the fancy stuff at the beginning, just write what you plan to do. Once you do it, remove the log message or keep it if it still makes sense.
Debug weird outcomes - you might expect to get a result set with multiple records, but are you checking to make sure you don’t get 0 records or null?
Write meaningful error messages - instead of “oops.” you might write
console.log("Oops: \n” + JSON.stringify(error,null,2));
to show you the contents of the error.
A quick example using Dad Jokes
Here’s a codepen example that takes a search term, calls the Icanhazdadjoke.com API, and returns some results. For clarity, we’re including both the first joke and the full response below.
When you get a successful request, you get a joke!
If you’d like to try it out, run the codepen, first searching for: astronaut, and then for Console.log. You’ll get a result like the one below when you click the Console option in Codepen.
How are we using Console.log?
We’re doing a few different things here in our console.log() calls.
First, we’re echoing the logic of what we’re doing:
console.log(`asking API for a joke about ${topic}`);
Using the backtick character lets us include some information about what was passed from our form using a POST and shows the topic that the user entered into the form. If we had used a “ character we would have gotten the string literal “asking API for a joke about ${topic}” instead of replacing the variable topic
with astronaut.
Second, we can send some information about what we received, namely the length of the array in the result set. This tells us how many jokes we received from the API.
console.log(`retrieved ${response.data.results.length} results`);
Finally, we need to note when we didn’t find a result and also got an error.
console.log("Didn't find a joke: \n" + JSON.stringify(error,null,2));
By using the JSON.stringify command, we would see the full result. If you want to trigger this failure, update the codepen that calls the API to a different URL that doesn’t resolve. You’ll get an error like this one.
Because we’ve trapped for a null result, the only way we would typically receive this error with the code in our sample codepen is if the API stopped responding.
What’s the takeaway? Console.log() is an important tool in your coding playbook. It’s a key way to echo what’s happening in code (even if the code seems relatively simple) and solve your issues faster. Adding a few key facts from your code to the output of the console makes it easier to debug later as well.
Links for Reading and Sharing
These are links that caught my 👀
1/ You can talk to GPT4V - ChatGPT now supports input through audio and still image (I’d guess it might also be able to handle video soon). If you’d like to see what you can say and show to GPT4V, here’s a summary.
2/ It’s the Spirit Halloween time of year - Spirit Halloween is not only the source of loud orange signs at empty store spaces in your local mall, but also a giant popup business. Trung Phan breaks it down and explains how they manage to cram an entire year’s revenue into about six weeks.
3/ What is Data UX? - Timo Dechau points out some specific ways we can measure data user experience. It doesn’t just happen in apps. Data UX is also about code formatting, field naming, and the way we design dashboards to be consistent.
What to do next
Hit reply if you’ve got links to share, data stories, or want to say hello.
Want to book a discovery call to talk about how we can work together?
The next big thing always starts out being dismissed as a “toy.” - Chris Dixon